Building with the Match API - Part 1 Address Search
6 Minute ReadThe Addresscloud Match API is a service that provides geocoding and reverse-geocoding. In this, the first of a series of posts I'm going to demonstrate using the API to perform an address search from a web page. The example also describes a multi-match workflow to query a list of addresses by postcode. All the code examples shown can be found in the Addresscloud tutorials repository on GitHub.
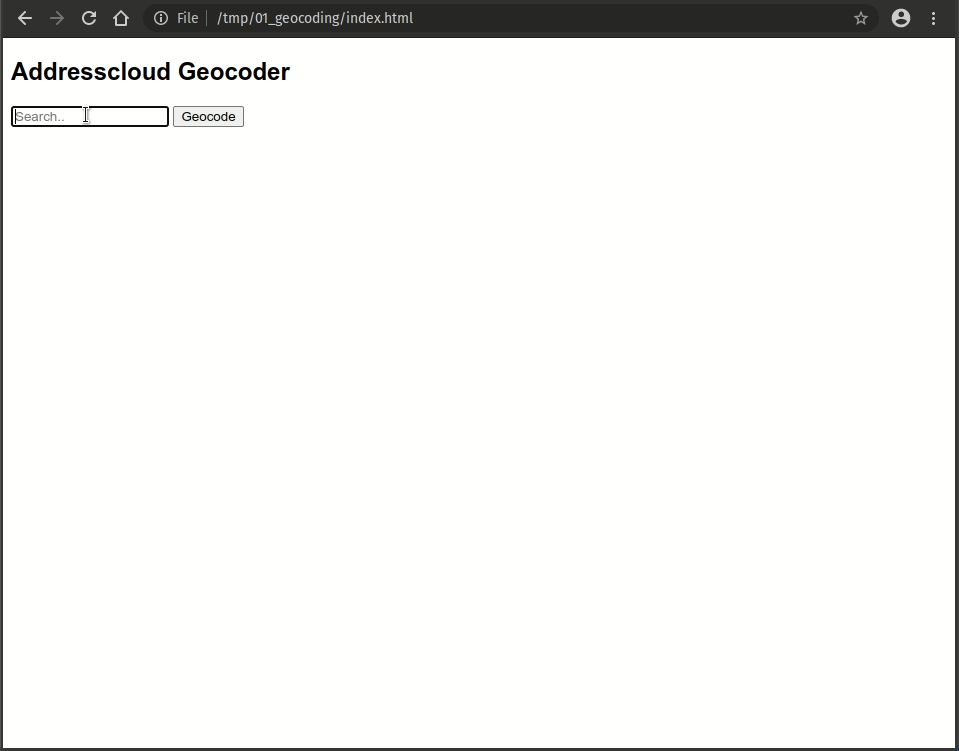
If you'd like an Addresscloud API key for testing please email support@addresscloud.com to request trial details.
Quickstart
- Download the tutorials repository from here.
- Unzip the folder and navigate to the workspace for this tutorial: interactive/match/01_geocoding.
- Add your Addresscloud API credentials to api.js and open index.html.
Workspace Overview
The workspace contains four files. The key files are index.html and api.js - the other files a responsible for the search box, button and drop-down list.
File | Description |
---|---|
css/app.css | stylesheet |
js/api.js | requests to Addresscloud |
js/app.js | web page logic |
index.html | web page |
Understanding api.js
The requests to Addresscloud's Match API can be found in api.js which uses the axios library to make API requests.
If you haven't done so already add your API credentials to the headers object on line four of api.js.
// Create axios instance
const api = axios.create({
baseURL: 'https://api.addresscloud.com/match/v1/address',
headers: { 'X-CLIENT-ID': 'my-client-id', 'X-API-KEY': 'my-api-key' }
})
// Geocode an address
async function geocode(address) {
const { data } = await api.get(`/geocode?query=${address}`)
return data
}
// Lookup a known address by Id
async function lookupById(id) {
const { data } = await api.get(`/lookup/byId/${id}`)
return data
}
As all the examples in this tutorial are working at address level, we've included the 'address' target in the base URL on line two. Addresscloud allows you to make requests against targets at different geographic scales (e.g. postcode and street). Further details on targets can be found at docs.addresscloud.com.
api.js contains two functions to perform the API requests:
- geocode() which calls the geocode endpoint
- lookupById() which calls the lookup endpoint for multi-match queries. The multi-match workflow is discussed more below.
At this point you should be able to open index.html and perform address searches against the Addresscloud Match API. When Addresscloud finds an address its properties are displayed in a table including coordinates, and other fields such as UPRN and UDRPN for British addresses.
In the example below there is no ambiguity in the address and the Match API returns an exact match. If you open your browser's developer console you can see the entire JSON object, including the match status attribute and the address properties which are displayed on the web page. To help developers the Addresscloud documentation contains full examples of responses from different queries for each endpoint.
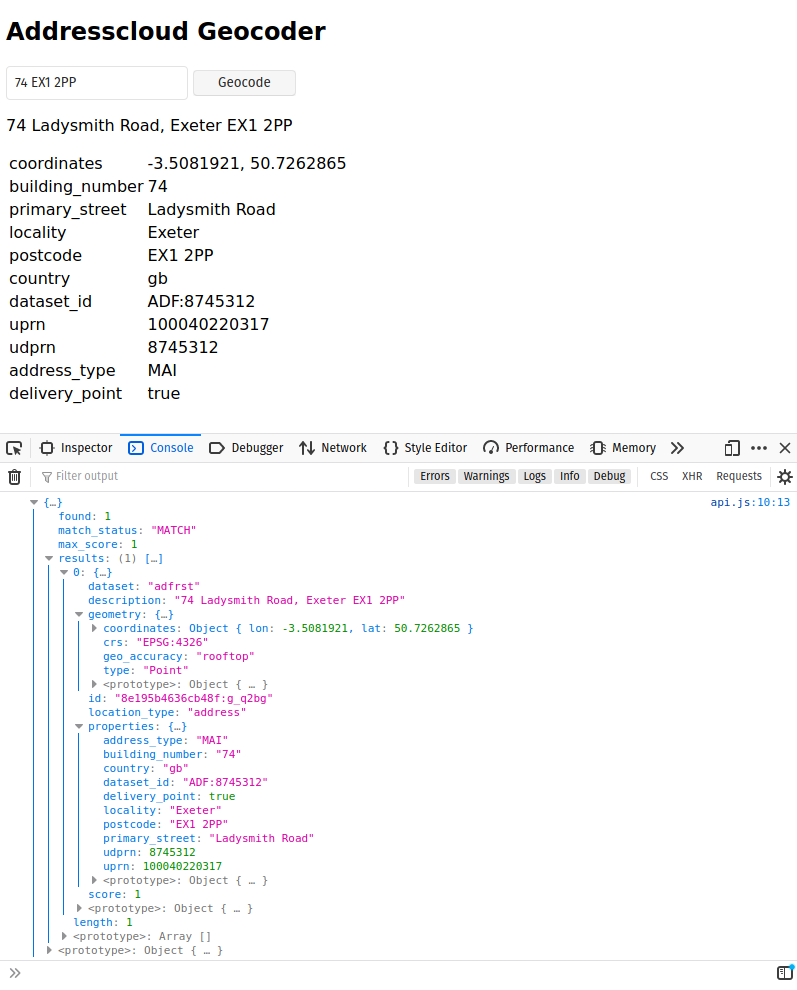
Multi-match Logic
When multiple addresses are found (e.g. when searching by postcode) the Match API returns a multi-match response with a "pick list" of Addresses. The pick list can then be displayed to the user for them to select the most appropriate, and a second call is made to the lookup-by-Id endpoint to get the details of the address. Selecting an address by its identifier is faster than submitting a second geocoding request. Its also worth noting that multi-match responses are non-billable, and only the final lookup-by-Id request counts towards your usage.
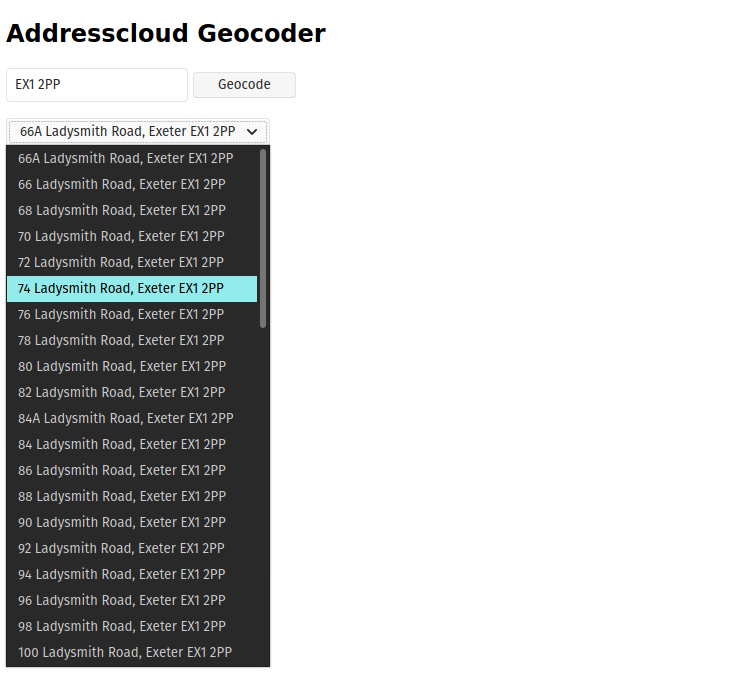
To determine whether a request has returned an exact match or a multi-match developers can interrogate the "found" property which shows a count of addresses found. The "match_status" parameter also returns either a "MATCH" or "MULTIMATCH" attribute.
{
"match_status": "MULTIMATCH",
"found": 53,
"max_score": 1,
"results": [
{
"dataset": "adfrst",
"location_type": "address",
"id": "8e195b4636ca297:v-yLUP",
"description": "66A Ladysmith Road, Exeter EX1 2PP",
"score": 1
},
// results continue....
{
"dataset": "adfrst",
"location_type": "address",
"id": "8e195b445993daf:frkEPF",
"description": "147 Ladysmith Road, Exeter EX1 2PP",
"score": 1
}
]
}
Referring to the search() function in app.js you can see a simple test against the "found" parameter to determine whether or not a pick list should be generated.
if (data.found === 1 ) {
setOutput(data.results[0])
} else {
createPicklist(data.results)
}
The createPicklist() function in app.js binds the lookup() function to the pick list. This means that when a user selects an address from the list its identifier is passed to the lookupById() function so the full record can be requested from Addresscloud and displayed on the page.
// Create a picklits of addresses
function createPicklist(list) {
let output = `<p><select id="picklist" onChange="lookup(this.value)">`
for (result of list) {
output += `<option value=${result.id}>${result.description}</option>`
}
output += `</select></p>`
document.getElementById('results').innerHTML = output
}
// Handle picklist lookups
async function lookup(id) {
const data = await lookupById(id)
setOutput(data.result)
}
Summary
In this tutorial we've built a simple address search and geocoding interface using the Addresscloud Match API. We've also added support for multi-match queries, returning pick lists which is a common use case in Great Britain for postcode searches. In the next instalment we'll take a look at adding a map to the web page to display address locations.
Addresscloud in Action
See how Addresscloud services are helping our customers solve real-world challenges.