Building with the Match API - Part 3 Reverse Geocoding
3 Minute Read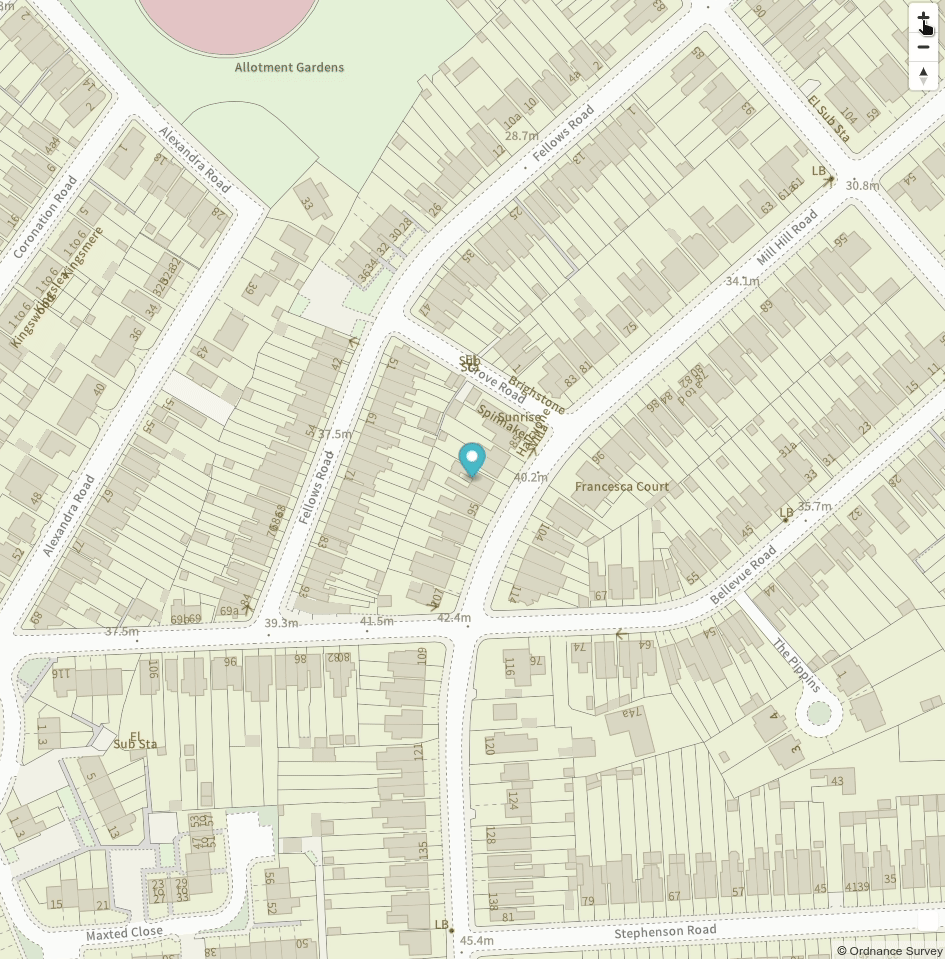
This tutorial builds on the material covered in Part 1 and Part 2. If you'd like an Addresscloud API key for testing please email support@addresscloud.com. All tutorials are available at https://github.com/addresscloud/tutorials.
Need an "out the box" solution? Addresscloud provides a fully-fledged and globally-available map service to its customers for property searches and risk assessments. Contact sales@addresscloud.com for more details.
Quickstart
- Download the tutorial repository from GitHub here.
- Unzip the folder and navigate to the workspace for this tutorial: interactive/match/03_reverse_geocoding.
- Add your Addresscloud API credentials to api.js.
- Add your OS Vector Tile API key (created in Part 2) to map.js
- Open index.html.
Configuring the API
Open js/api.js and add your Addresscloud API key to the axios instance. Similar to Part 2, the reverseGeocode() function calls the Addresscloud geoode endpoint. However, instead of passing an address string into the query object a latitude/longitude pair is passed into the coordinates query. The endpoint also takes a search radius parameter (metres) for the reverse geocode search. Your api.js file should look as follows:
// Setup
const api = axios.create({
baseURL: 'https://api.addresscloud.com/match/v1/address',
headers: { 'X-CLIENT-ID': 'client-id', 'X-API-KEY': 'api-key' }
})
// Geocode an address
async function reverseGeocode(lon, lat, radius) {
const { data } = await api.get(`/geocode?coordinates=${lon},${lat}&radius=${radius}`)
return data
}
Building the Map
Add your OS API key at the top of the js/map.js file to enable the OS Vector tiles layer. The map works by allowing the user to drag a marker around, and once the user releases the marker the coordinates are captured and sent to the reverseGeocode() function. This functionality is handled by the onDragEnd() function, which displays the reverse geocoding results in a pop-up.
async function onDragEnd() {
var { lng, lat } = marker.getLngLat();
const data = await reverseGeocode(lng, lat, 5)
document.getElementById('address').innerHTML = `<h2>Address</h2>`
for (const address of data.results){
document.getElementById('address').innerHTML += `<h3>${address.description}</h3>`
}
console.log(address)
}
marker.on('dragend', onDragEnd);
Testing
Once you've updated your API keys you should be able to view index.html to see the map. Drop the pointer on a building to see its address! This simple example shows how you can quickly get setup to perform precise reverse geocoding requests, using Addresscloud's geocoding API. In the next tutorial we'll extend the service to add data from Addresscloud's intel service, including property attributes and geographic risk data.
Addresscloud in Action
See how Addresscloud services are helping our customers solve real-world challenges.